What is Chip-8 Programming Language?
What is Emulator?
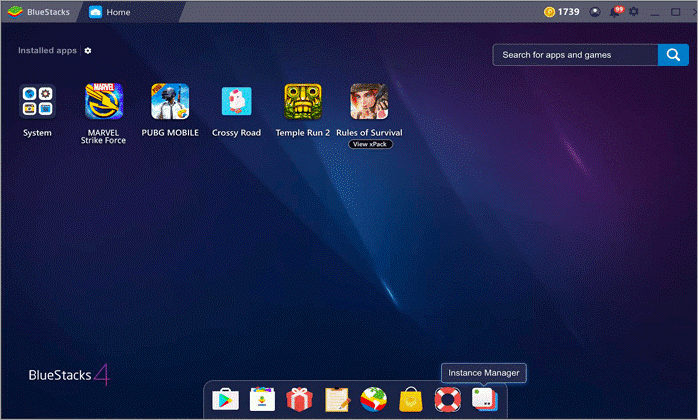
How to write an Emulator?

Game Loop
#include #include // OpenGL graphics and input #include “chip8.h” // Your cpu core implementation chip8 myChip8; int main(int argc, char **argv) { // Set up render system and register input callbacks setupGraphics(); setupInput(); // Initialize the Chip8 system and load the game into the memory myChip8.initialize(); myChip8.loadGame(“pong”); // Emulation loop for(;;) { // Emulate one cycle myChip8.emulateCycle(); // If the draw flag is set, update the screen if(myChip8.drawFlag) drawGraphics(); // Store key press state (Press and Release) myChip8.setKeys(); } return 0; }
Emulation cycle
void chip8::initialize() { // Initialize registers and memory once } void chip8::emulateCycle() { // Fetch Opcode // Decode Opcode // Execute Opcode // Update timers }
CHIP-8 Opcode table
Installation
Go install github.com/bradford-hamilton/chippy